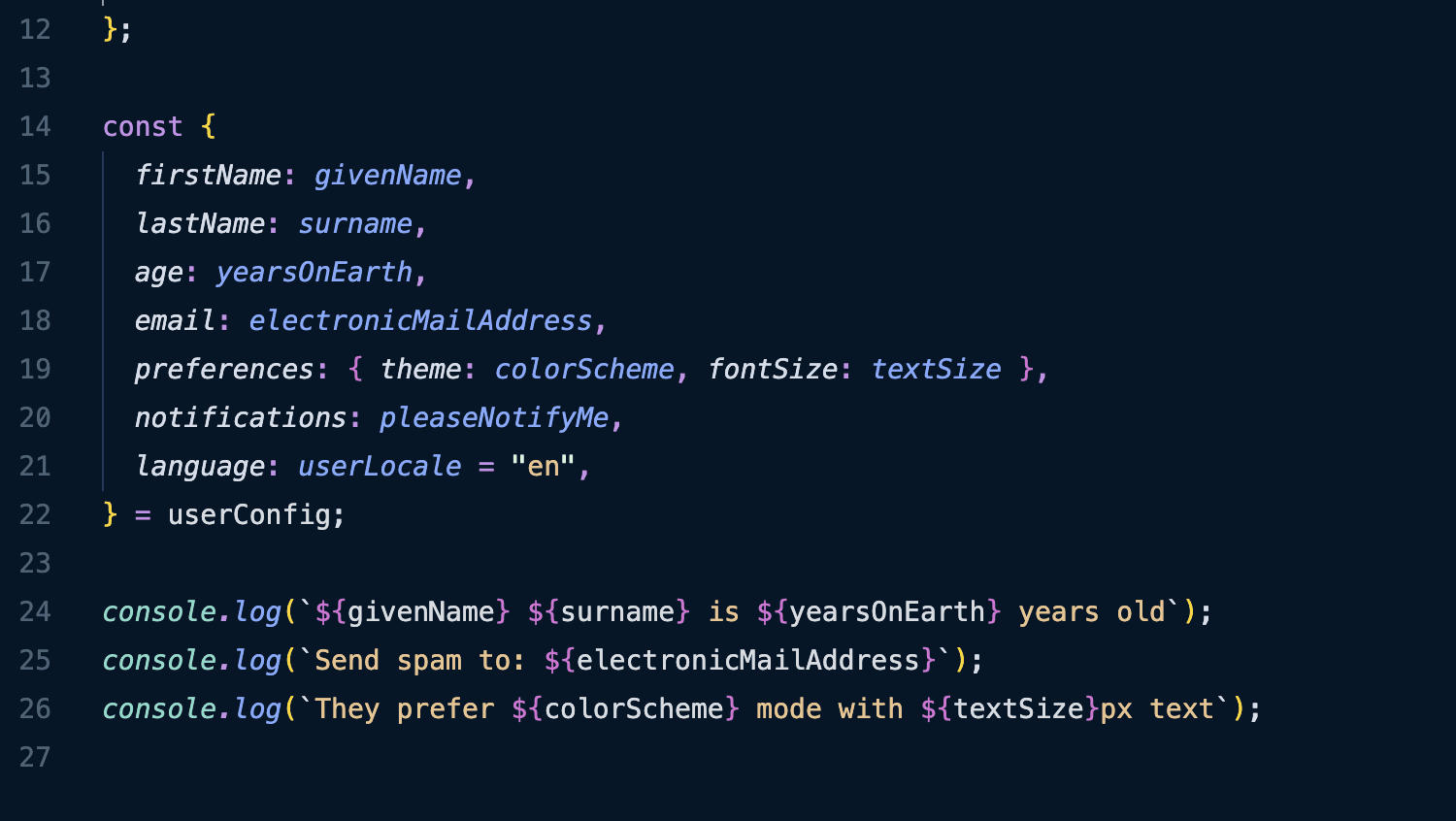
Utilizing Destructuring Assignment in JavaScript and React
Object destructuring assignment in JavaScript is a crucial feature. Destructuring assignment refers to the ability to extract specific properties from an object and treat them as new variables.
Let's consider a specific example of extracting information from a user profile. First, let's define a user profile object:
let userProfile = {
name: "Satoshi Tajiri",
email: "[email protected]",
age: 58,
location: "Tokyo",
};
Without destructuring assignment, you would extract each property like this:
let name = userProfile.name;
let email = userProfile.email;
let age = userProfile.age;
let location = userProfile.location;
However, with destructuring assignment, you can extract these properties in a single line:
let { name, email, age, location } = userProfile;
This code extracts the name
, email
, age
, and location
properties from the user profile object and assigns them to new variables with the same names.
Now, let's utilize this in React components. In React, it's common to destructure component properties (props). This makes the code cleaner and each property more explicit. Using the same user profile display example as before, let's first define a component to display the user profile:
function UserProfile(props) {
return (
<div>
<h1>{props.name}</h1>
<p>Email: {props.email}</p>
<p>Age: {props.age}</p>
<p>Location: {props.location}</p>
</div>
);
}
In this component, we're directly referencing props to extract each property. However, using destructuring assignment, we can write it more simply:
function UserProfile({ name, email, age, location }) {
return (
<div>
<h1>{name}</h1>
<p>Email: {email}</p>
<p>Age: {age}</p>
<p>Location: {location}</p>
</div>
);
}
In the code above, we're destructuring the props object in the function's parameter section. As a result, we no longer need to repeat props.
throughout the code, and we can access properties more intuitively.
Destructuring assignment is an effective tool for making code concise and improving readability. By understanding this fundamental JavaScript feature and applying it to React development, you can write more efficient and readable code.
This article was originally published on Qiita.